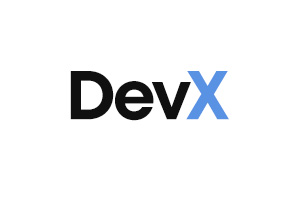
Retrieving a file from a jar file
JAR file in Java is a compressed format and is used for packaging of the deliverables. At times, you may want to manipulate this file.Below example indicates a scenario for the same. import java.util.jar.*;import java.io.*; public class RetrievingJarEntry{   public static void main(String args[])   {      RetrievingJarEntry retrievingJarEntry = new RetrievingJarEntry();      retrievingJarEntry.proceed();   }      private void proceed()   {      String sourceJarFile = “files/contacts.jar”;      String sourceFile = “2.txt”;      String destFile = “files/new2.txt”;      try{                  JarFile jarFile = new JarFile(sourceJarFile);         JarEntry jarEntry = jarFile.getJarEntry(sourceFile);         System.out.println(“Found entry: ” + jarEntry);         if ( jarEntry != null)         {            //Getting the jarEntry into the inputStream            InputStream inputStream = jarFile.getInputStream(jarEntry);             //Creating a output stream to a new file of our choice            FileOutputStream fileOutputStream = new java.io.FileOutputStream(destFile);            System.out.println(“Attempting to create file: ” + destFile);            while (inputStream.available()  0)             {                 fileOutputStream.write(inputStream.read());            }            System.out.println(“Created file: ” + destFile);            fileOutputStream.close();            inputStream.close();         }      }catch(IOException ioe)      {         System.out.println(“Exception: ” + ioe);      }   }} /* Expected output: [root@mypc]# java RetrievingJarEntryFound entry: 2.txtAttempting to create file: files/new2.txtCreated file: files/new2.txt */ //Please note: You have to create a folder with name files and a jar file contacts.jar which has files 1.txt, 2.txt and 3.txt